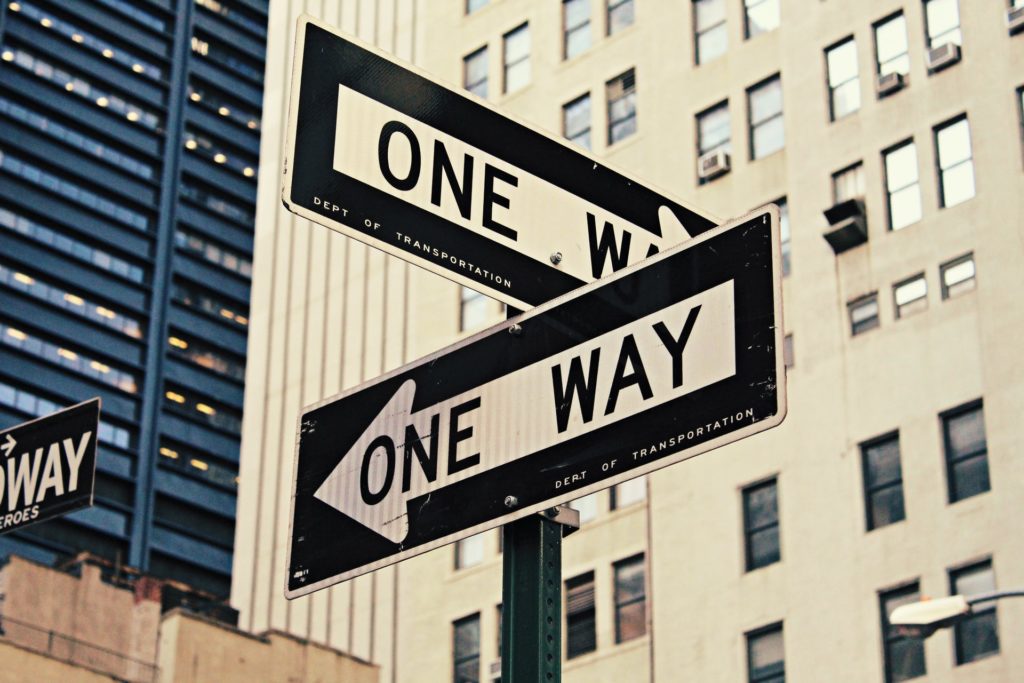
tl;dr
Arrow functions are very easy and I’m just an old man.
I remember that I saw arrow functions in js for the first time. It was pretty hard to understand for me, but I didn’t do anything in js in years, so I just get used to this unknown.
But recently I’m working in a project with PHP 7.4. And guess what. Yup, arrow functions arrived in PHP in exactly this version. So I hadn’t a choice but to sit down and understand this little bastard.
Arrow functions in PHP are fairly simple, yet quite nice, and a little different than ordinary lambda functions.
Example
For given dataset:
$data = [
[
'name' => 'John',
'surname' => 'Smith',
'gender' => 'm',
],
[
'name' => 'Pocahontas',
'surname' => 'N/A',
'gender' => 'w',
],
];
Let’s say that we want to filter woman from $data.
Using lambda:
array_filter(
$data,
function (array $person) {
return 'w' === $person['gender'];
}
);
Using arrow functions:
array_filter(
$data,
fn(array $person) => return 'w' === $person['gender']
);
Fairly simple, huh? I don’t know what gave me this difficulty, maybe I spent too much time using older PHP versions 🤷♂️
So, what are the differences?
1. oneliners
Lambda functions in PHP can have as many lines as you want (it’s maybe not the best idea to make them too long though). But not arrow functions. They can only be oneliners. Probably because of parsing engine or so.
2. access to variables outside the scope
If you want to use variables outside a lambda function you have to use use ()
syntax, for example:
$someFlag = false;
array_filter(
$data,
function (array $person) use ($someFlag) {
return $someFlag ? 'w' === $person['gender'] : 'm' === $person['gender'];
}
);
But in arrow functions you have access to all variables inside parent’s scope:
$someFlag = false;
array_filter(
$data,
fn(array $person) => return $someFlag ? 'w' === $person['gender'] : 'm' === $person['gender'];
);
Little, but handy thing.
Simple tip(?)
Recently I saw usage of arrow function like so:
fn($_, int $value) => return ++$value;
This $_
was puzzling me. But when I dig deeper I saw that closure where this function was used has 2 parameters: key and value. So $_
was only a placeholder for the unused key. Any other name could be used here, but using $_
is very clever, because it looks just like placeholder for something not used.
Leave a Reply